Introduction To Version Control With Git
1. Version Control
Version control is the practice of tracking changes to any kind of data like code, documents, or artwork. Version control systems provide a complete change history and allow versions of the same data to be manipulated separately.
2. Git
The entire change history is kept in git repositories. Git repositories can be manipulated on your personal computer or shared with others on websites like GitLab.
3. Git Basics
3.1. Initializing the Repository
Setting up repository, git init. Ensure git is installed. How to install Git.
git -v
The repository is .git. To initialize the repository do:
git init
3.2. Version Control With Git
Changes must be staged and committed in the same directory as the repository to be tracked by git. The contents of the next commit are determined by what changes are staged. git commit creates a new snapshot of the staged changed.
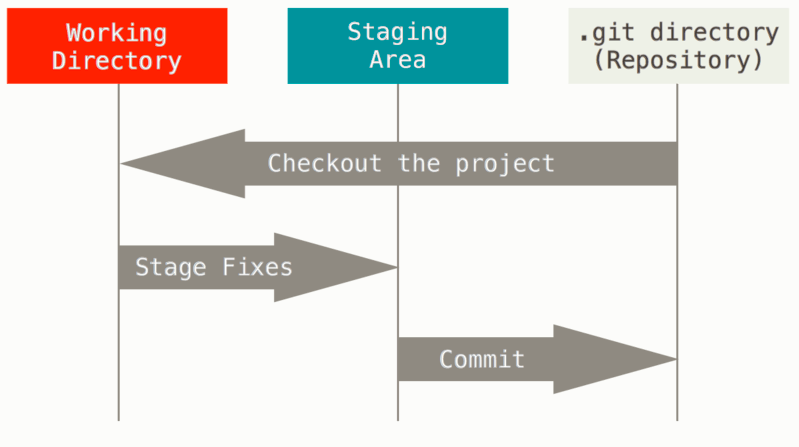
3.3. git add
The .git file stages changes. The next commit is described by the staged changes. git add stages the latest changes of whatever file it is targeting. s
git add SuperImportantPaper.txt
git add .
3.4. git commit
git commit appends a new snapshot, specified by the staged changes, to the git repository.
To track information about the commit author, git needs and email and name.
git config user.email "AllFather@Highlands.Above"
git config user.name "YourAllFather"
You need to specify the email and name for commits to the repository, otherwise the following error occurs when running git commit.
$ git commit
Author identity unknown
*** Please tell me who you are.
Run
git config --global user.email "you@example.com"
git config --global user.name "Your Name"
to set your account's default identity.
Omit --global to set the identity only in this repository.
fatal: unable to auto-detect email address
git commit
Enter a commit message with the default text editor set for git commit.
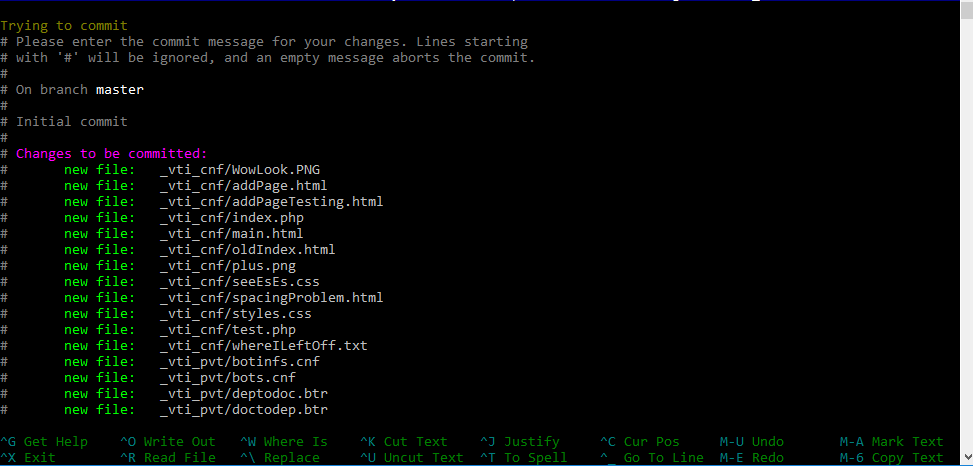
3.5. Branches
A branch is a independent series of commits. Each repository begins with a main /master branch. Branching can be used to keep a separate series of commits from the main to facilitate multiple people working on the same set of data.
$ git branch -v
# A fancy way to see the branches with git log. Press q to quit
$ alias.lgb=log --graph --pretty=format:'%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr) %C(bold blue)<%an>%Creset%n' --abbrev-commit --date=relative --branches
$ git lgb
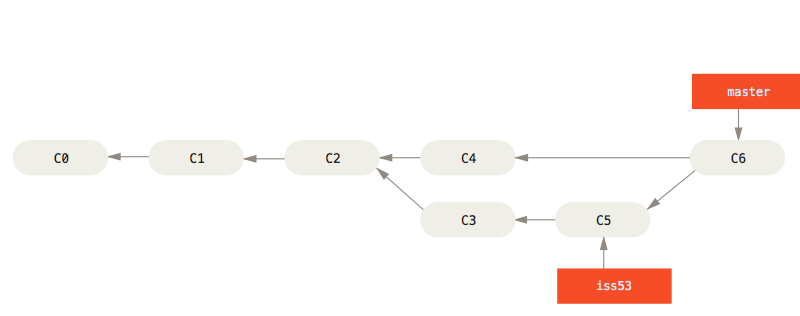
3.6. git branch
git branch starts a separate branch from the current branch. Changes to this branch won’t be tracked in other branches.
$ git branch differentBranch
$ git branch -v
4. Hosted Git Basics
-
GitHub and GitLab are online platforms used to collaborate and **
-
store repositories. ** To edit a repository stored on GitHub or GitLab it must be cloned. GitHub and GitLab provide forking capabilities which are similar to branching.
4.1. Clone A Repository
git clone allows editing of a repository on GitLab. git clone downloads the repository from GitLab into the current working directory.
To clone a repository navigate to it’s page on GitLab and copy it’s clone link:
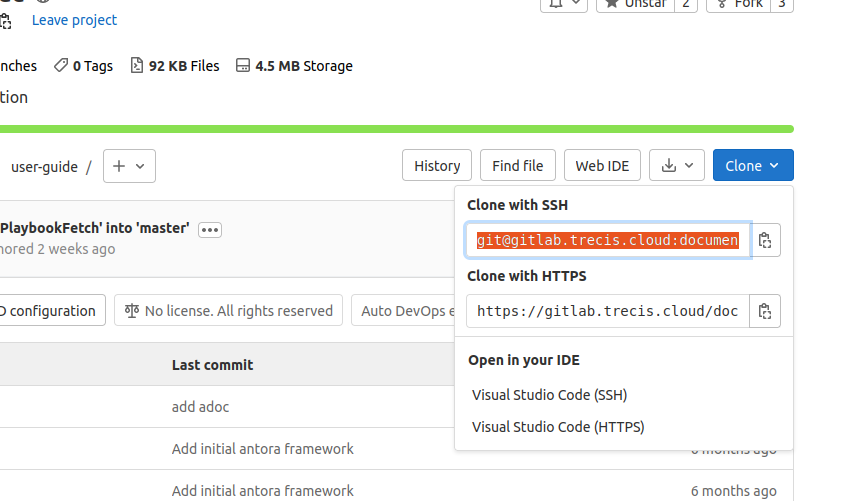
$ git clone git@gitlab.trecis.cloud:nbc170001/docs-science-support-meeting-minutes.git
4.2. Push To A Repository
git push allows users to make changes to the repository stored on GitLab. git push uploads the local snapshot of the repository to the online repository.
$ git push origin yourBranch
4.3. Fork and Pull A Repository
Forking allows users to make their own copy of another person’s repository on GitLab which they can freely edit.
To fork a repository navigate to the repository on GitLab and select fork:
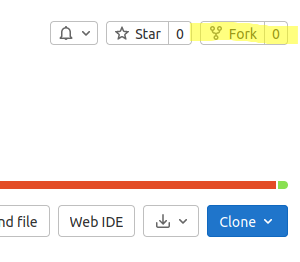
To make changes to your fork, clone the repository and push your changes.
Pulling is how changes are made to a GitLab repository based on it’s fork.
To submit a pull request navigate to the original repository’s GitLab page and do compare and pull request button:
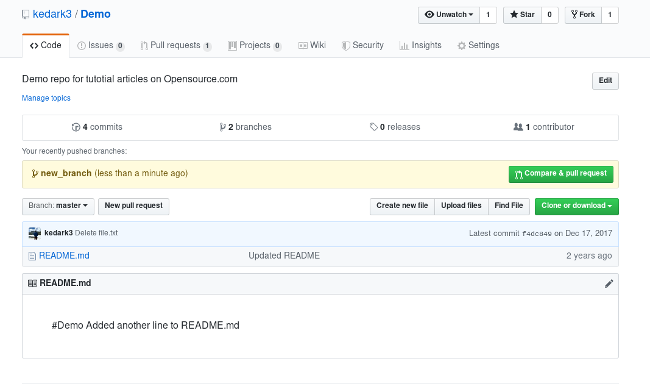
References
-
[issue] https://gitlab.trecis.cloud/documentation/user-guide/-/issues/11
-
[gitscm] https://git-scm.com/book/en/v2/Getting-Started-About-Version-Control
-
[gitscm2] https://git-scm.com/book/en/v2/Getting-Started-What-is-Git%3F
-
[gitscm3] https://git-scm.com/book/en/v2/Getting-Started-Installing-Git
-
[gitscm4] https://git-scm.com/book/en/v2/Git-Branching-Basic-Branching-and-Merging
-
[atlassian] https://www.atlassian.com/git/tutorials/what-is-version-control
-
[atlassian2] https://www.atlassian.com/git/tutorials/using-branches/git-merge
-
[overflow] https://stackoverflow.com/questions/7022890/how-to-display-the-tag-name-and-branch-name-using-git-log-graph/7023272